#1 INSERT์ ๋ฐํ๊ฐ
[Android] Room - Entity, DAO, Database
#1 ์ด์ ๊ธ [Android] Room - ๊ธฐ์ด, INSERT์ DELETE ์ฐ์ต # Room ์๊ฐ Room์ ์ฌ์ฉํ์ฌ ๋ก์ปฌ ๋ฐ์ดํฐ๋ฒ ์ด์ค์ ๋ฐ์ดํฐ ์ ์ฅ | Android Developers Room ๋ผ์ด๋ธ๋ฌ๋ฆฌ๋ฅผ ์ฌ์ฉํ์ฌ ๋ ์ฝ๊ฒ ๋ฐ์ดํฐ๋ฅผ ์ ์งํ๋ ๋ฐฉ๋ฒ ์์๋ณด
kenel.tistory.com
Room์ DAO์ ์ด๋ค ๋ฉ์๋๋ค์ Long ๋๋ List<Long>ํ return์ ๊ฐ์ง ์ ์๋ค (์ ๊ฒ์๊ธ์ #5-1 ์ฐธ์กฐ). ์๋์ ์๋ ์ํ ์ฑ์ ์์ ํ์ฌ @Insert ์ด๋ ธํ ์ด์ ์ด ๋ถ์ ๋ฉ์๋์ return์ Long์ผ๋ก ๋๊ณ , ํด๋น return์ ํ๋ฉด์ ํ์ํ๊ฒ ๋ง๋ค์ด๋ณธ๋ค.
#2 ์์ ํ ์ํ ์ฑ
[Android] Room - ๊ธฐ์ด, INSERT์ DELETE ์ฐ์ต
#1 Room ์๊ฐ Room์ ์ฌ์ฉํ์ฌ ๋ก์ปฌ ๋ฐ์ดํฐ๋ฒ ์ด์ค์ ๋ฐ์ดํฐ ์ ์ฅ | Android Developers Room ๋ผ์ด๋ธ๋ฌ๋ฆฌ๋ฅผ ์ฌ์ฉํ์ฌ ๋ ์ฝ๊ฒ ๋ฐ์ดํฐ๋ฅผ ์ ์งํ๋ ๋ฐฉ๋ฒ ์์๋ณด๊ธฐ developer.android.com SQLite๋ ๋ชจ๋ฐ์ผ ๊ธฐ๊ธฐ๋ฅผ ์ํ
kenel.tistory.com
์ ๊ฒ์๊ธ์ ์์ฑ๋ ์ฑ์ ๊ธฐ๋ฐ์ผ๋ก ์์ ์ ๊ฐํ๋ค. "Save" ๋ฒํผ์ ๋๋ฅผ ์, INSERT๋ Entity์ ๊ธฐ๋ณธํค id๊ฐ์ Toast ๋ฉ์์ง๋ก ์ถ๋ ฅํ๊ฒ ๋ง๋ค์ด๋ณด๊ฒ ๋ค.
#3 ์ฝ๋ ์์
#3-1 ๊ฐ์
[Android] ViewModel - View์ Event ๋ฐ์์ํค๊ธฐ
#1 ์ํ ์ฑ [Android] ViewModel - View์ ๊ฐ์ฒด(ViewModel) ์ ๋ฌ#1 ๊ฐ์ #1-1 Data Binding๊ณผ ViewModel [Android] Data Binding - View์ ๊ฐ์ฒด ์ ๋ฌ #1 ๊ฐ์ฒด ์ ๋ฌ์ ํ์์ฑ #1-1 ์ด์ ๊ธ Data Binding - ๊ธฐ์ด #1 ๋ฐ์ดํฐ ๋ฐ์ธ๋ฉ ์ฌ์ฉ
kenel.tistory.com
View์ Toast ๋ฉ์์ง๋ฅผ ํ์ํ๋ ์์ ์ ๊ตฌํํ๊ธฐ ์ํด, #3-3์ #3-4๋ ์ ๊ฒ์๊ธ์ ๊ธฐ๋ฐํ์ฌ ์์ ๋์๋ค.
#3-2 Repository (UserRepository.kt) ์์
// package com.example.insertionresponse.db
class UserRepository(private val dao: UserDAO) {
...
suspend fun insert2(user: User): Long {
return dao.insertUser2(user)
}
...
}
#3-3 ViewModel (UserViewModel.kt) ์์
...
class UserViewModel(private val repository: UserRepository) : ViewModel() {
...
private val _toastMessage = MutableLiveData<Event<String>>()
val toastMessage: LiveData<Event<String>>
get() = _toastMessage
init {
...
}
// ์ด๊ธฐํ ๊ด๋ จ
...
// ๋ฒํผ ํด๋ฆญ ์ ๋์
fun saveOrUpdate() {
if (isUpdateOrDelete) {
// Update ์์
TODO()
} else {
// Save ์์
insert2(inputtedUser.value!!)
clearInputBox()
}
}
...
// Repository ๋์๊ณผ ๊ด๋ จ๋ ๋ฉ์๋๋ค
...
fun insert2(user: User) = viewModelScope.launch(Dispatchers.IO) {
val insertedId = repository.insert2(user)
withContext(Dispatchers.Main) { // LiveData์ ๊ฐ ๋ณ๊ฒฝ์ ์ค๊ณ ์ Main ์ค๋ ๋์์๋ง ์คํ๋์ด์ผ ํจ
if (-1 < insertedId) {
_toastMessage.value = Event("Inserted Id is $insertedId")
} else {
_toastMessage.value = Event("Insertion failed")
}
}
}
...
// ๊ธฐํ UI ๋ค๋ฌ๊ธฐ์ฉ ๋ฉ์๋๋ค
...
}
#3-4 Activity (MainActivity.kt) ์์
...
class MainActivity : AppCompatActivity() {
...
override fun onCreate(savedInstanceState: Bundle?) {
...
displayToastEvent()
}
...
private fun displayToastEvent() {
userViewModel.toastMessage.observe(this, Observer {
it.getContentIfNotHandled()?.let { // Only proceed if the event has never been handled
Toast.makeText(this, it, Toast.LENGTH_SHORT).show()
}
})
}
}
#4 ์๋ ํ์ธ
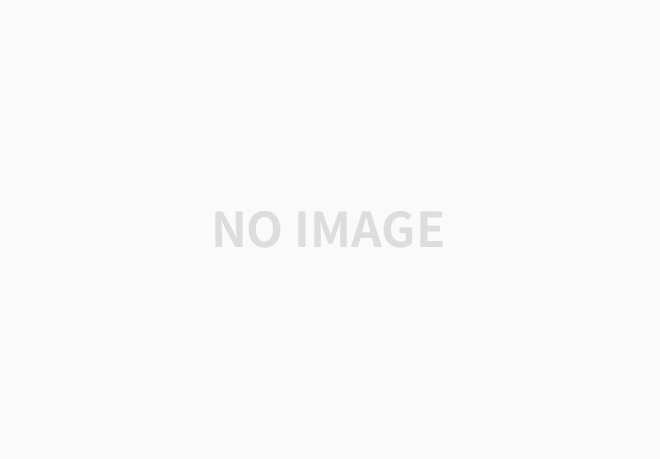
#5 ์์ฑ๋ ์ฑ
android-practice/room/InsertionResponse at master ยท Kanmanemone/android-practice
Contribute to Kanmanemone/android-practice development by creating an account on GitHub.
github.com
'๊นจ์ ๊ฐ๋ ๐ > Android' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[Android] Room - AutoMigrationSpec (0) | 2024.05.08 |
---|---|
[Android] Room - AutoMigration ๊ธฐ์ด (0) | 2024.05.06 |
[Android] RecyclerView - notifyDataSetChanged() (0) | 2024.02.28 |
[Android] ViewModel - View์ Event ๋ฐ์์ํค๊ธฐ (0) | 2024.02.27 |
[Android] Room - UPDATE ์ฐ์ต (0) | 2024.02.26 |